This is part 2 of 2. In part 1 I characterize what is meant by "database application". I also quickly review some different types of regression testing, and then describe a hypothetical retail inventory example system which will serve as the subject of detailed discussion. In part 2, I explore the "final table" type of regression test in detail, and apply it to our retail inventory system. I conclude with an argument for why "final table" testing should always be included on your project.
The challenge
I’m the project manager for the AIR system, which was described in some detail in part 1. The system has been humming along in production for years, and now my developers are working on some significant functional changes to the system which are scheduled to be released shortly. My biggest concern is to not break something that is already working. For that I need some type of regression testing, but I"m unsure what the best regression strategy is. Some, but not all, of my system is already covered by Unit tests, and there are a couple of higher level functional tests that cover a slice. But there is no end-to-end fully integrated systems test.
In an ideal (fantasy) world, I would now instruct my developers to write regression tests, at all levels, covering every aspect of the system. They would write low level unit tests to test every DB function, DB stored procedure, and Java method. They would then write a higher level functional test for each slice. Finally, they would script, or automate, a highest level fully integrated end-to-end systems test.
Figures 1 & 2 (same as in part 1) diagram the system.
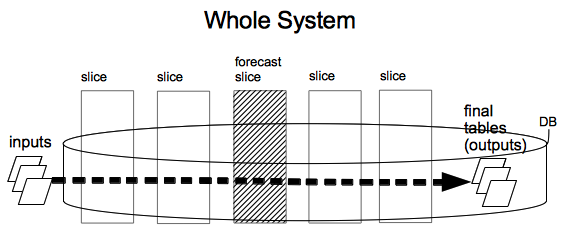
Figure 1: System
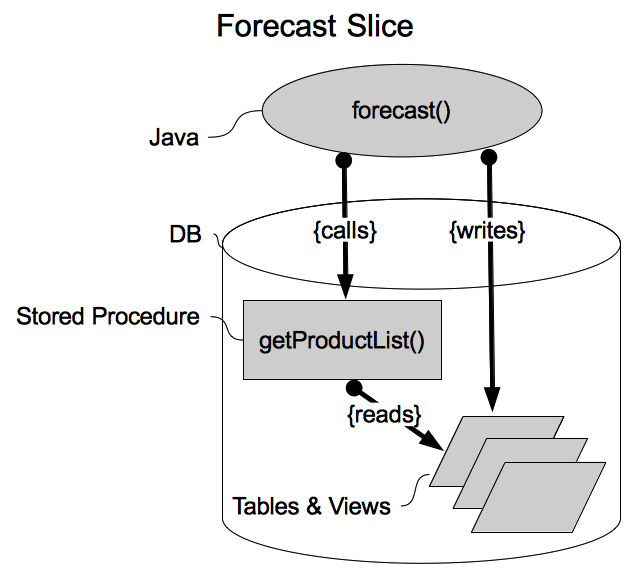
Figure 2: Forecast slice
Taking the Forecast slice as an example, if I want to competely cover this slice with Unit tests, my developers will need to write several for the getProductList() stored procedure. More than one Unit will be needed in order to exercises all of the possible boundary and error conditions. The test harness will need to somehow populate the underlying tables/views that are read by getProductList(). Just the Unit tests required to provide decent coverage for the getProductList() could take an entire day to write. Next, my Java developers need to provide Units to blanket the forecast() method. Since forecast() calls the getProductList() stored procedure, the forecast() Units will need to mock the stored procedure. Setting up mock stored procedures is itself an exacting and laborious endeavour. Again, providing comprehensive Unit coverage for just forecast() might pin down a Java developer for an entire day. Finally, all of the tables and views touched by this slice will also need their own Unit tests. These Unit tests will ensure that defaults, invariants, and constraints are always observed within the tables and views. If the views implement any business logic, that too must be covered by Unit testing.
All of the above Unit tests are data intensive. So in each case, the test writer must think long and hard about the possible range of data values that the subject function might be exposed to. The test writer will need to think about both expected values for each parameter or data set, and also possible values for each. After all, one of the goals of software testing is to ensure that the test subject continues to operate properly even in the face of unexpected or "flaky" data or paramters.
It’s clear that providing deep Unit test coverage for just the Forecast slice will entail significant effort. 3 developer days (1 for java, 1 for stored procedure, and 1 for tables/views) might be generous, but it’s not an unrealistic estimate, considering all of the different concerns involved. The Forecast slice is just one of many slices that constitute the whole system. In a complex enterprise system, and that includes most Big Enterprise systems, there will be hundreds or thousands of slices.
If my whole system has just 100 slices, it will take 300 developer days to achieve exquisite, comprehensive, rock-solid, Unit test coverage. Current software testing theory dictates that this is exactly the type of Unit testing you should have on a well run project. But as the manager of project AIR, I don’t have 300 developer days to write Unit tests. Purists will object that most of the Unit tests should already have been written before the current iteration, but on project AIR (as on many, if not most, Enterprise systems) they weren’t. That’s the fault of the previous project manager, but he has since moved on, so now it’s my problem. The theory dictates perfect Unit testing, but it’s hard to square that with time and resource constraints:
In theory there is no difference between theory and practice. In practice there is.
Jan L. A. van de Snepscheut
Alternatively, I can abandon the idea of blanket Unit test coverage. Instead, I could go to the other extreme and build just 1, fully integrated, end-to-end, systems test. One way to carry out this system test is to:
Final Table Test
- capture a complete set of inputs (files, tables, whatever) from the current production (pre-change) system.
- in an isolated, controlled, test environment, run those inputs through the complete current production (pre-change) system in order to produce outputs. I’ll call the outputs "final tables", because all of the outputs are captured in DB tables and those tables are at the end of a chain (or pipeline) of processing. The outputs from this run are labelled "expected".
- in an isolated, controlled, test environment, run those same inputs through the complete current in-development (post-change) system in order to produce outputs. Label those outputs "actual".
- compare the expected final tables with the actual final tables. They should match, except in the areas where there are intended functional changes that are meant to produce changes to the outputs.
Some benefits of this scheme are worth considering carefully. This is the ulimately "black box" test. The testers do not need to understand anything at all about the internals of the system. They only need to know which buttons to press in order to run the system, and which are the "final tables" they need to compare. So this is the type of test that an independent testing group (say QA) should be able to effect. Another interesting aspect of this approach is that of test data selection. The good part is that it relies on current production inputs, exactly the real-world data conditions that the application will experience in practice. Also, simply reusing production data alleviates the burden of the test team needing to carefully consider and synthesize test data. This could be a significant labor savings.
But perhaps the most important aspect of this type of testing is that it effectively tests the system at all levels. Put another way, the final table test can provide much of the test coverage that would be provided by the collection of Units, whereas Units cannot provide any coverage at the functional or system levels. As a result, even if you have a blanket of Unit tests, you still really do need to do some form of functional or systems test. That’s because the interfaces, or boundaries, between functions, modules, and slices have to be exercised. As an example, look at the Forecast Slice. Suppose I write individual Unit tests for getProductList(), forecast(), and the tables and views. I test the forecast() method by mocking up the getProductList(). That’s not sufficient to provide confidence that the slice is production ready. I need at least some functional tests that exercise forecast() making a real call against the getProductList() stored procedure. Otherwise, the Unit tests, in mocking the getProductList(), might make assumptions about the parameters, behaviour, or side effects, that are not consistent with the real (non-mock) getProductList(). Likewise, it’s not enough for me to simply write a functional test that just covers each slice. I need a still higher level test that exercises the interfaces between slices. So the bottom line is that, if you absolutely have to, you can live without Unit tests and rely exclusively on a single, integrated, systems test (final table test). But the converse is not true. Even if you have superbe Unit test coverage, you will still need to perform some integrated systems testing.
Of course, the final table test is hardly bulletproof. Probably the biggest problem with this test is that it is a relative test, wherease the Units are absolute. By that I mean the final table test only tells you that the changed system behaves the same as the reference (current production) system that you compare it against. If the current production system is producing incorrect results, and your changed system passes the final table test, then your changed system will also be producing incorrect results in exactly the same fashion. The Unit tests, on the other hand, are measured against absolute expectations, not relative ones. So Unit tests are better grounded. Also, the final table test only tests aspects of the system that will be exercised by the current production data inputs. It’s possible that there are bugs lurking in the newly developed (changed) system, but that the bugs are not triggered by the current production data inputs. But the production data could change at any time in a way that would then trigger the bug, but in production. One more material drawback of the final table test is that it can only be carried out late in the Software Development Cycle, whereas Unit tests are able to uncover problems very early on in the cycle. That’s important because it’s generally, though not always, more expensive to correct bugs late in the cycle than early in the cycle.
Units | Final Table | |
---|---|---|
bug detection | early | late |
level | lowest, function | highest, system |
test type | white box | black box |
coverage | isolated | comprehensive |
results type | absolute | relative |
effort | large | small |
impl. expertise | programming | operations |
data | synthetic | production |
The key to deriving maximum benefit from the final table test is that the final tables comparison be carried out at the actual field value level. That is, every row+column in the expected final tables must be compared with its corresponding row+column in the actual final tables. Remarkably, very few shops that utilize a fully integrated systems test actually carry out these detailed comparisons. Instead, they rely on gross (summary level) comparisons, and visual inspection of the resulting data sets. That is completely inadequate.Many bugs can, and do, elude gross and visual comparisons.
The conclusion is that you must perform some type of fully integrated systems test, and a very powerful and cost effective option for this is the "final table test". Of course, high quality projects will also have low-level Unit tests and mid-level functional tests. However, if you are in a bind, constrained by very limited developer and testing resources, I would recommend that the first and foremost test you should carry out is the final table test.
There are lots of information about latest technology and how to get trained in them, like Big Data Training Chennai have spread around the web, but this is a unique one according to me. The strategy you have updated here will make me to get trained in future technologies. By the way you are running a great blog. Thanks for sharing this.
ReplyDeleteCloud storage is a model of data storage where the digital data is stored in logical pools, the physical storage spans multiple servers (and often locations), and the physical environment is typically owned and managed by a hosting company. The way you have explained everything is quite impressive and elegant. Thank you so much for sharing this data in here.
ReplyDeletecloud computing training in chennai
cloud computing training
cloud computing training chennai
ReplyDeleteThanks for sharing this valuable information to our vision. You have posted a trust worthy blog keep sharing.
Regards,
SAP training in chennai|sas training in Chennai|SAP course in chennai
Quite Interesting post!!! Thanks for posting such a useful post. I wish to read your upcoming blog to enhance my skill set, keep blogging.
ReplyDeleteRegards,
Informatica course in Chennai|Informatica training in chennai|Informatica courses in Chennai
Excellant post!!!. The strategy you have posted on this technology helped me to get into the next level and had lot of information in it.
ReplyDeleteSAS Training in Chennai | SAS Course in Chennai
Nice Post! It is really interesting to read from the beginning & I would like to share your blog to my circles, keep your blog as updated.
ReplyDeleteRegards,
JAVA Training in Chennai|JAVA Course in Chennai|PHP Training in Chennai
ReplyDeleteThe strategy you posted was nice. The people who want to shift their career to the IT sector then it is the right option to go with the ethical hacking course.
Ethical hacking course in Chennai | Ethical hacking training in chennai
The post about regression testing is very much helpful.Thanks for sharing it
ReplyDeleteSelenium Training Center in Chennai
The post about regression testing is very much helpful.Thanks for sharing it
ReplyDeleteSelenium Training Center in Chennai
coach outlet
ReplyDeleteconverse shoes
ysl outlet
birkenstock sandals
uggs
the north face outlet
oakley vault sunglasses
rolex watches
michael kors handbags
longchamp bags
20161124caiyan
coach factory outlet
ReplyDeletetoms outlet
fitflops
christian louboutin outlet
hollister clothing
ugg sale
coach outlet store
mlb jerseys wholesale
pandora charms
fitflops shoes
20170109
toms shoes
ReplyDeletenike air max
mcm outlet
parker pens
new balance sneakers
replica rolex watches
nike free running shoes
michael kors handbags
michael kors handbags
christian louboutin shoes
2017.3.21xukaimin
I appreciate this work amazing post for us I like it. I must say we should have an online discussion on this. We also share some information about our business
ReplyDeleteeCommerce Support Services In India
You have provided an nice article, Thank you very much for this one. And i hope this will be useful for many people.. and i am waiting for your next post keep on updating these kinds of knowledgeable things...
ReplyDeleteOnline Marketing Services
Top SEO Company in Bangalore
seo pricing india
Thank you for excellent article.
ReplyDeletePlease refer below if you are looking for best project center in coimbatore
final year projects in coimbatore
Spoken English Training in coimbatore
final year projects for CSE in coimbatore
final year projects for IT in coimbatore
final year projects for ECE in coimbatore
final year projects for EEE in coimbatore
final year projects for Mechanical in coimbatore
final year projects for Instrumentation in coimbatore
Good job! Fruitful article. I like this very much. It is very useful for my research. It shows your interest in this topic very well. I hope you will post some more information about the software. Please keep sharing!!
ReplyDeleteGerman Classes in Chennai
German Language Classes in Chennai
Hadoop Training in Chennai
Python Training in Chennai
German classes in OMR
German classes in Porur
German classes in Tnagar
best software testing institute in chennai
ReplyDeleteSoftware Testing Course in Chennai
Useful post regarding database technology
ReplyDeleteBest Data Science training in Chennai
I feel satisfied to read your blog, you have been delivering a useful & unique information to our vision.keep blogging.
ReplyDeleteRegards,
PHP Training in Chennai
PHP Course in Chennai
Tally Course in Chennai
ReactJS Training in Chennai
gst classes in chennai
ui design course in chennai
microsoft dynamics crm training in chennai
PHP Training in Chennai
PHP Course in Chennai
GOOD POST....
ReplyDeleteSelenium Testing Internship
Linux Internship
C Internship
CPP Internship
Embedded System Internship
Matlab Internship
nice
ReplyDeleteGeteventlisteners javascript
Karl fischer titration interview questions
How to hack tp link router
T system aptitude questions
Resume for bca final year student
Test case for railway reservation system
T systems pune placement papers
Infrrd bangalore interview questions
Max number in javascript
Paypal integration in php step by step pdf
NICE....
ReplyDeleteFREE Internship in Nagpur For Computer Engineering Students
Internship For MCA Students
Final Year Projects For Information Technology
Web Design Class
Mechanical Engineering Internship Certificate
Inplant Training For Mechanical Engineering Students
Inplant Training Certificate
Ethical Hacking Course in Chennai
Winter Internship For ECE Students
Internships For ECE Students in Bangalore
good
ReplyDeletehacking course
internship for it students
civil engineering internship report pdf india
ccna course chennai
internship report for civil engineering students in india
internships in hyderabad for cse students 2018
kashi infotech
cse internships in hyderabad
inplant training for diploma students
internship in hyderabad for cse students
good
ReplyDeletenodejs while loop
icici bank po interview questions and answers pdf
craterzone aptitude test
zensoft recruitment process
java developer resume 1 years experience
python developer resume pdf
infrrd private limited interview questions
js int max value
delete * from table oracle
t systems pune aptitude questions
good
ReplyDeleteResume Format For Bca Freshers
British Airways Interview Questions And Answers Pdf
Asus Tf101 Android 8
Crome://Flags/
T Systems Aptitude Test
Python Resume Ror 2 Years Experience
Ajax Redirect To Another Page With Post Data
Paramatrix Technologies Aptitude Questions And Answers
Adder Subtractor Comparator Using Ic 741 Op-Amp Theory
How To Hack Wifi With Ubuntu
I would like to thank you for the efforts you have made in writing this article.
ReplyDeleteSelenium Training in chennai | Selenium Training in anna nagar | Selenium Training in omr | Selenium Training in porur | Selenium Training in tambaram | Selenium Training in velachery
Diversify - It may be tempting to focus all of your media promotion on one site such as Facebook, but if you really want to supercharge your results, then you need to establish a presence on multiple social media sites, which will allow you to reach as much of your target audience as possible. keep itu p guys
ReplyDeleteAi & Artificial Intelligence Course in Chennai
PHP Training in Chennai
Ethical Hacking Course in Chennai Blue Prism Training in Chennai
UiPath Training in Chennai
ReplyDeleteเล่นเกมแล้วได้เงิน ได้ง่ายๆ ฟรีเครดิต สล็อตออนไลน์ เล่นสล็อต slot ไม่มีโกง คลิกเลยที่นี่
https://www.slot2xl.com/
I liked this blog.. I got some clear information from this blog.. Thanks for taking a time to share this blog...
ReplyDeleteEthical Hacking Course in Porur
Ethical Hacking Course in OMR
Ethical Hacking Course in Tambaram
Ethical Hacking Course in Anna Nagar
Ethical Hacking Course in T Nagar
Really, it’s a useful blog. Thanks for sharing this information.
ReplyDeleteIonic Online Course
Kotlin Online Course
social media marketing Online Training
React Native Online Training
R programming Training in Chennai
Xamarin Course in Chennai
Ionic Course in Chennai
supreme outlet
ReplyDeletecurry 6 shoes
air jordan
yeezy boost 350 v2
hermes handbags
supreme hoodie
moncler
hermes
balenciaga shoes
yeezy supply
Nice blog, Thanks for sharing this useful information.
ReplyDeleteMobile Application Testing Online Training
Mobile App Development Online Course
Mobile App Development Courses in Chennai
Google Analytics Online Course
Google Analytics Training in Chennai
Content Writing Course in Chennai
Online Content Writing Course
Really, it’s a useful blog. Thanks for sharing this information.
ReplyDeleteIonic Online Course
Kotlin Online Course
social media marketing Online Training
React Native Online Training
Xamarin Online Course
React native Online Course
เกมสล็อตออนไลน์แจกหนักแจกจริง พร้อมระบบฝากถอนอัตโนมัติบริการ 24 ชั่วโมง จาก pgslot
ReplyDeleteมีเกมให้เลือกเล่นมากกว่าร้อยเกม
ทั้งสล็ตอออนไลน์และยิงปลาออนไลน์ แค่สมัครสมาชิกก็รับ โบนัสสล็อตเครดิตฟรี ได้เลย
Good Job! It is the best explanation for this title. Well Done..
ReplyDeleteSales Training in Chennai
Sales Course in Chennai
SharePoint Training in Chennai
Wow! Excellent blog and I really impressed. Keep doing...
ReplyDeleteUi Ux Design Course in Chennai
Ui Ux Course in Chennai
UiPath Online Training
UiPath Training in Chennai
UiPath Training in Bangalore
เล่นเกมแล้วได้เงิน สล็อตออนไลน์ pgslot เล่นง่ายๆ เล่นสล็อต ฟรีเครดิต
ReplyDeleteได้เงินจริง เล่นเลยที่นี่ คลิกเลย เกมยิงปลา อย่าพลาดมาลุ้นกันเลย slotxo
https://www.slot4u.com
The best online slots website.
ReplyDeleteสล็อตเว็บตรง
The best online slots website.
ReplyDeletePGSLOT
The best online slots website.
ReplyDeleteสล็อตออนไลน์
Good article for everyone. Thank for good article
ReplyDeleteหวยออนไลน์
This is a great informative post. I appreciate your efforts and the valuable information you’ve provided. Keep up the good work!
ReplyDeletewine store